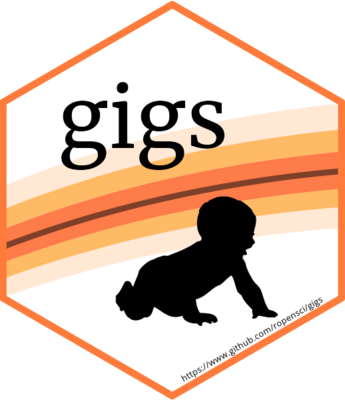
Classify wasting in data.frame
-like objects with GIGS-recommended growth standards
Source: R/growth_classify.R
classify_wasting.Rd
Classify wasting in data.frame
-like objects with GIGS-recommended growth
standards
Usage
classify_wasting(
.data,
weight_kg,
lenht_cm,
age_days,
gest_days,
sex,
id = NULL,
.new = c("wlz", "wasting", "wasting_outliers")
)
Arguments
- .data
A
data.frame
-like tabular object with one or more rows. Must be permissible bycheckmate::assert_data_frame()
, so you can also supply atibble
,data.table
, or similar.- weight_kg
<
data-masking
> The name of a column in.data
which is a numeric vector of birth weight values in kg. It is assumed that weight measurements provided to this function are birth weights recorded <12 hours after an infant's birth.- lenht_cm
<
data-masking
> The name of a column in.data
which is a numeric vector of length/height values in cm.- age_days
<
data-masking
> The name of a column in.data
which is a numeric vector of age values in days.- gest_days
<
data-masking
> The name of a column in.data
which is a numeric vector of gestational age(s) at birth in days. This column, in conjunction with the column referred to byage_days
, is used to select which growth standard to use for each observation.- sex
<
data-masking
> The name of a column in.data
which is a case-sensitive character vector of sexes, either"M"
(male) or"F"
(female). By default, gigs will warn you if any elements ofsex
are not"M"
or"F"
, or are missing (NA
). You can customise this behaviour using the GIGS package-level options.- id
<
data-masking
> The name of a column in.data
which is a factor variable with IDs for each observation. When notNULL
, this variable is used to ensure that only the first measurement taken from each infant is used as a birth measure. If all your data is from one individual, leave this parameter asNULL
. Default =NULL
.- .new
A three-length character vector with names for the output columns. These inputs will be repaired if necessary using
vctrs::vec_as_names()
, which will print any changes to the console. If any elements in.new
are the same as elements incolnames(.data)
, the function will throw an error. Default =c("wlz", "wasting", "wasting_outliers")
.
Value
A tabular object of the same class that was provided as .data
,
with three new columns named according to .new
. These columns will be
(from left to right):
wlz
- Numeric vector of weight-for-length/height zscoreswasting
- Factor of wasting categories without outlier flaggingwasting_outliers
- Factor of wasting categories with outlier flagging
Details
Cut-offs for wasting categories are:
Category | Factor level | Z-score bounds |
Severe wasting | "wasting_severe" | wlz =< -3 |
Wasting | "wasting" | -3 < wlz =< -2 |
No wasting | "not_wasting" | abs(wlz) < 2 |
Overweight | "overweight" | wlz >= 2 |
Outlier | "outlier" | abs(wlz) > 5 |
Note
Categorical (factor) columns produced here may contain unused factor
levels. By default, gigs will inform you if these columns have unused
factor levels. You can change this behaviour using the
GIGS package-level option
.gigs_options$handle_unused_levels
.
References
'Implausible z-score values' in World Health Organization (ed.) Recommendations for data collection, analysis and reporting on anthropometric indicators in children under 5 years old. Geneva: World Health Organization and the United Nations Children's Fund UNICEF, (2019). pp. 64-65.
'Percentage of children stunted, wasted, and underweight, and mean z-scores for stunting, wasting and underweight' in Guide to DHS Statistics DHS-7 Rockville, Maryland, USA: ICF (2020). pp. 431-435. https://dhsprogram.com/data/Guide-to-DHS-Statistics/Nutritional_Status.htm
See also
See classify_growth()
to run this analysis and others at the same
time. See gigs_waz()
to learn which growth standard will be used for
different combinations of gestational/chronological age.
Examples
# This dummy dataset contains data from two people, from birth (<3 days) to
# 500 days of age.
data <- data.frame(
child = factor(rep.int(c("A", "B"), c(3, 3))),
agedays = c(0, 100, 500, 2, 100, 500),
gestage = c(rep(35 * 7, 3), rep(35 * 7, 3)),
sex = rep.int(c("M", "F"), c(3, 3)),
weight_kg = c(3, 6, 9, 3, 6, 9),
length_cm = rep.int(c(52.2, 60.4, 75), 2)
)
# Use the `id` argument to ensure that `classify_wasting` uses the correct
# standard for each observation
data |>
classify_wasting(weight_kg = weight_kg,
lenht_cm = length_cm,
age_days = agedays,
gest_days = gestage,
sex = sex,
id = child)
#> Warning: There was 1 'at birth' observation where `age_days` > 0.5.
#> ℹ This occurred for ID B.
#> ! Unused factor levels kept after wasting categorisation: "wasting_severe",
#> "wasting", and "overweight".
#> ! Unused factor levels kept after wasting categorisation: "wasting_severe",
#> "wasting", "overweight", and "outlier".
#> child agedays gestage sex weight_kg length_cm wlz wasting
#> 1 A 0 245 M 3 52.2 NA <NA>
#> 2 A 100 245 M 6 60.4 1.6408675 not_wasting
#> 3 A 500 245 M 9 75.0 -0.6621871 not_wasting
#> 4 B 2 245 F 3 52.2 NA <NA>
#> 5 B 100 245 F 6 60.4 1.3314150 not_wasting
#> 6 B 500 245 F 9 75.0 -0.1829182 not_wasting
#> wasting_outliers
#> 1 <NA>
#> 2 not_wasting
#> 3 not_wasting
#> 4 <NA>
#> 5 not_wasting
#> 6 not_wasting
# If you don't specify `id`, `classify_wasting` will assume data is from one
# child only
data |>
classify_wasting(weight_kg = weight_kg,
lenht_cm = length_cm,
age_days = agedays,
gest_days = gestage,
sex = sex)
#> ! Unused factor levels kept after wasting categorisation: "wasting_severe" and
#> "overweight".
#> ! Unused factor levels kept after wasting categorisation: "wasting_severe",
#> "overweight", and "outlier".
#> child agedays gestage sex weight_kg length_cm wlz wasting
#> 1 A 0 245 M 3 52.2 NA <NA>
#> 2 A 100 245 M 6 60.4 1.6408675 not_wasting
#> 3 A 500 245 M 9 75.0 -0.6621871 not_wasting
#> 4 B 2 245 F 3 52.2 -2.3716265 wasting
#> 5 B 100 245 F 6 60.4 1.3314150 not_wasting
#> 6 B 500 245 F 9 75.0 -0.1829182 not_wasting
#> wasting_outliers
#> 1 <NA>
#> 2 not_wasting
#> 3 not_wasting
#> 4 wasting
#> 5 not_wasting
#> 6 not_wasting