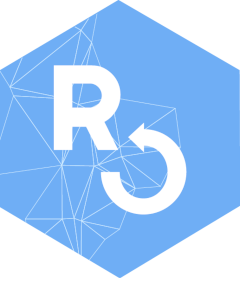
Create a gist via git instead of the GitHub Gists HTTP API
Source:R/gist_create_git.R
gist_create_git.Rd
Create a gist via git instead of the GitHub Gists HTTP API
Arguments
- files
Files to upload. this or
code
param must be passed- description
(character) Brief description of gist (optional)
- public
(logical) Whether gist is public (default: TRUE)
- browse
(logical) To open newly create gist in default browser (default: TRUE)
- knit
(logical) Knit code before posting as a gist? If the file has a
.Rmd
or.Rnw
extension, we run the file withknit
, and if it has a.R
extension, then we userender
- code
Pass in any set of code. This can be a single R object, or many lines of code wrapped in quotes, then curly brackets (see examples below). this or
files
param must be passed- filename
Name of the file to create, only used if
code
parameter is used. Default tocode.R
- knitopts, renderopts
- include_source
(logical) Only applies if
knit=TRUE
. Include source file in the gist in addition to the knitted output.- artifacts
(logical/character) Include artifacts or not. If
TRUE
, includes all artifacts. Or you can pass in a file extension to only upload artifacts of certain file exensions. Default:FALSE
- imgur_inject
(logical) Inject
imgur_upload
into your.Rmd
file to upload files to https://imgur.com/. This will be ignored if the file is a sweave/latex file because the rendered pdf can't be uploaded anyway. Default: FALSE- git_method
(character) One of ssh (default) or https. If a remote already exists, we use that remote, and this parameter is ignored.
- sleep
(integer) Seconds to sleep after creating gist, but before collecting metadata on the gist. If uploading a lot of stuff, you may want to set this to a higher value, otherwise, you may not get accurate metadata for your gist. You can of course always refresh afterwards by calling
gist
with your gist id.- ...
Further args passed on to
verb-POST
Details
Note that when browse=TRUE
there is a slight delay in when
we open up the gist in your default browser and when the data will display
in the gist. We could have this function sleep a while and guess when it
will be ready, but instead we open your gist right after we're done sending
the data to GitHub. Make sure to refresh the page if you don't see your
content right away.
Likewise, the object that is returned from this function call may not have
the updated and correct file information. You can retrieve that easily by
calling gist()
with the gist id.
This function uses git instead of the HTTP API, and thus requires
the R package git2r
. If you don't have git2r
installed, and
try to use this function, it will stop and tell you to install git2r
.
This function using git is better suited than gist_create()
for use cases involving:
Big files - The GitHub API allows only files of up to 1 MB in size. Using git we can get around that limit.
Binary files - Often artifacts created are binary files like
.png
. The GitHub API doesn't allow transport of binary files, but we can do that with git.
Another difference between this function and gist_create()
is
that this function can collect all artifacts coming out of a knit process.
If a gist is somehow deleted, or the remote changes, when you try to push
to the same gist again, everything should be fine. We now use
tryCatch
on the push attempt, and if it fails, we'll add a new
remote (which means a new gist), and push again.
Examples
if (FALSE) { # \dontrun{
# prepare a directory and a file
unlink("~/gitgist", recursive = TRUE)
dir.create("~/gitgist")
file <- system.file("examples", "stuff.md", package = "gistr")
writeLines(readLines(file), con = "~/gitgist/stuff.md")
# create a gist
gist_create_git(files = "~/gitgist/stuff.md")
## more than one file can be passed in
unlink("~/gitgist2", recursive = TRUE)
dir.create("~/gitgist2")
file.copy(file, "~/gitgist2/")
cat("hello world", file = "~/gitgist2/hello_world.md")
list.files("~/gitgist2")
gist_create_git(c("~/gitgist2/stuff.md", "~/gitgist2/hello_world.md"))
# Include all files in a directory
unlink("~/gitgist3", recursive = TRUE)
dir.create("~/gitgist3")
cat("foo bar", file="~/gitgist3/foobar.txt")
cat("hello", file="~/gitgist3/hello.txt")
list.files("~/gitgist3")
gist_create_git("~/gitgist3")
# binary files
png <- system.file("examples", "file.png", package = "gistr")
unlink("~/gitgist4", recursive = TRUE)
dir.create("~/gitgist4")
file.copy(png, "~/gitgist4/")
list.files("~/gitgist4")
gist_create_git(files = "~/gitgist4/file.png")
# knit files first, then push up
# note: by default we don't upload images, but you can do that,
# see next example
rmd <- system.file("examples", "plots.Rmd", package = "gistr")
unlink("~/gitgist5", recursive = TRUE)
dir.create("~/gitgist5")
file.copy(rmd, "~/gitgist5/")
list.files("~/gitgist5")
gist_create_git("~/gitgist5/plots.Rmd", knit = TRUE)
# collect all/any artifacts from knitting process
arts <- system.file("examples", "artifacts_eg1.Rmd", package = "gistr")
unlink("~/gitgist6", recursive = TRUE)
dir.create("~/gitgist6")
file.copy(arts, "~/gitgist6/")
list.files("~/gitgist6")
gist_create_git("~/gitgist6/artifacts_eg1.Rmd", knit = TRUE,
artifacts = TRUE)
# from a code block
gist_create_git(code={'
x <- letters
numbers <- runif(8)
numbers
[1] 0.3229318 0.5933054 0.7778408 0.3898947 0.1309717 0.7501378 0.3206379 0.3379005
'}, filename="my_cool_code.R")
# Use https instead of ssh
png <- system.file("examples", "file.png", package = "gistr")
unlink("~/gitgist7", recursive = TRUE)
dir.create("~/gitgist7")
file.copy(png, "~/gitgist7/")
list.files("~/gitgist7")
gist_create_git(files = "~/gitgist7/file.png", git_method = "https")
} # }