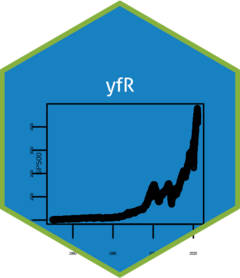
Transforms a long (stacked) data frame into a list of wide data frames
Source:R/yf_data_convert_to_wide.R
yf_convert_to_wide.Rd
Transforms a long (stacked) data frame into a list of wide data frames
Examples
my_f <- system.file("extdata/example_data_yfR.rds", package = "yfR")
df_tickers <- readRDS(my_f)
print(df_tickers)
#> # A tibble: 1,077 × 10
#> ticker ref_date price_open price_high price_low price_close price_adjusted
#> * <chr> <date> <dbl> <dbl> <dbl> <dbl> <dbl>
#> 1 ^BVSP 2020-01-02 115652 118573 115649 118573 118573
#> 2 ^BVSP 2020-01-03 118564 118792 117341 117707 117707
#> 3 ^BVSP 2020-01-06 117707 117707 116269 116878 116878
#> 4 ^BVSP 2020-01-07 116872 117076 115965 116662 116662
#> 5 ^BVSP 2020-01-08 116667 117335 115693 116247 116247
#> 6 ^BVSP 2020-01-09 116248 116820 115411 115947 115947
#> 7 ^BVSP 2020-01-10 115948 116745 114952 115503 115503
#> 8 ^BVSP 2020-01-13 115503 117333 115503 117325 117325
#> 9 ^BVSP 2020-01-14 117325 117705 116610 117632 117632
#> 10 ^BVSP 2020-01-15 117632 117632 116188 116414 116414
#> # ℹ 1,067 more rows
#> # ℹ 3 more variables: volume <dbl>, ret_adjusted_prices <dbl>,
#> # ret_closing_prices <dbl>
l_wide <- yf_convert_to_wide(df_tickers)
l_wide
#> $price_open
#> # A tibble: 369 × 4
#> ref_date `^BVSP` FB MMM
#> <date> <dbl> <dbl> <dbl>
#> 1 2020-01-02 115652 207. 178.
#> 2 2020-01-03 118564 207. 177.
#> 3 2020-01-06 117707 207. 177.
#> 4 2020-01-07 116872 213. 178.
#> 5 2020-01-08 116667 213 178
#> 6 2020-01-09 116248 218. 182.
#> 7 2020-01-10 115948 219. 182.
#> 8 2020-01-13 115503 220. 181.
#> 9 2020-01-14 117325 222. 181.
#> 10 2020-01-15 117632 221. 181.
#> # ℹ 359 more rows
#>
#> $price_high
#> # A tibble: 369 × 4
#> ref_date `^BVSP` FB MMM
#> <date> <dbl> <dbl> <dbl>
#> 1 2020-01-02 118573 210. 180.
#> 2 2020-01-03 118792 210. 179.
#> 3 2020-01-06 117707 213. 179.
#> 4 2020-01-07 117076 215. 179.
#> 5 2020-01-08 117335 216. 182.
#> 6 2020-01-09 116820 218. 182.
#> 7 2020-01-10 116745 220. 182.
#> 8 2020-01-13 117333 222. 182.
#> 9 2020-01-14 117705 222. 183.
#> 10 2020-01-15 117632 222. 182.
#> # ℹ 359 more rows
#>
#> $price_low
#> # A tibble: 369 × 4
#> ref_date `^BVSP` FB MMM
#> <date> <dbl> <dbl> <dbl>
#> 1 2020-01-02 115649 206. 177.
#> 2 2020-01-03 117341 207. 176.
#> 3 2020-01-06 116269 207. 176.
#> 4 2020-01-07 115965 212. 177.
#> 5 2020-01-08 115693 213. 178.
#> 6 2020-01-09 115411 216. 180.
#> 7 2020-01-10 114952 217. 180.
#> 8 2020-01-13 115503 219. 180.
#> 9 2020-01-14 116610 219. 180.
#> 10 2020-01-15 116188 220. 179.
#> # ℹ 359 more rows
#>
#> $price_close
#> # A tibble: 369 × 4
#> ref_date `^BVSP` FB MMM
#> <date> <dbl> <dbl> <dbl>
#> 1 2020-01-02 118573 210. 180
#> 2 2020-01-03 117707 209. 178.
#> 3 2020-01-06 116878 213. 179.
#> 4 2020-01-07 116662 213. 178.
#> 5 2020-01-08 116247 215. 181.
#> 6 2020-01-09 115947 218. 181.
#> 7 2020-01-10 115503 218. 180.
#> 8 2020-01-13 117325 222. 181.
#> 9 2020-01-14 117632 219. 181.
#> 10 2020-01-15 116414 221. 180.
#> # ℹ 359 more rows
#>
#> $price_adjusted
#> # A tibble: 369 × 4
#> ref_date `^BVSP` FB MMM
#> <date> <dbl> <dbl> <dbl>
#> 1 2020-01-02 118573 210. 171.
#> 2 2020-01-03 117707 209. 169.
#> 3 2020-01-06 116878 213. 170.
#> 4 2020-01-07 116662 213. 169.
#> 5 2020-01-08 116247 215. 171.
#> 6 2020-01-09 115947 218. 172.
#> 7 2020-01-10 115503 218. 171.
#> 8 2020-01-13 117325 222. 172.
#> 9 2020-01-14 117632 219. 172.
#> 10 2020-01-15 116414 221. 171.
#> # ℹ 359 more rows
#>
#> $volume
#> # A tibble: 369 × 4
#> ref_date `^BVSP` FB MMM
#> <date> <dbl> <dbl> <dbl>
#> 1 2020-01-02 5162700 12077100 3601700
#> 2 2020-01-03 6834500 11188400 2466900
#> 3 2020-01-06 6570000 17058900 1998000
#> 4 2020-01-07 4854100 14912400 2173000
#> 5 2020-01-08 5910500 13475000 2758300
#> 6 2020-01-09 5953500 12642800 2746300
#> 7 2020-01-10 4783400 12119400 2103800
#> 8 2020-01-13 5685100 14463400 2090800
#> 9 2020-01-14 5361700 13288900 2911200
#> 10 2020-01-15 5569400 10036500 2482200
#> # ℹ 359 more rows
#>
#> $ret_adjusted_prices
#> # A tibble: 369 × 4
#> ref_date `^BVSP` FB MMM
#> <date> <dbl> <dbl> <dbl>
#> 1 2020-01-02 NA NA NA
#> 2 2020-01-03 -0.00730 -0.00529 -0.00861
#> 3 2020-01-06 -0.00704 0.0188 0.000953
#> 4 2020-01-07 -0.00185 0.00216 -0.00403
#> 5 2020-01-08 -0.00356 0.0101 0.0153
#> 6 2020-01-09 -0.00258 0.0143 0.00316
#> 7 2020-01-10 -0.00383 -0.00110 -0.00403
#> 8 2020-01-13 0.0158 0.0177 0.00249
#> 9 2020-01-14 0.00262 -0.0128 0.00249
#> 10 2020-01-15 -0.0104 0.00954 -0.00877
#> # ℹ 359 more rows
#>
#> $ret_closing_prices
#> # A tibble: 369 × 4
#> ref_date `^BVSP` FB MMM
#> <date> <dbl> <dbl> <dbl>
#> 1 2020-01-02 NA NA NA
#> 2 2020-01-03 -0.00730 -0.00529 -0.00861
#> 3 2020-01-06 -0.00704 0.0188 0.000953
#> 4 2020-01-07 -0.00185 0.00216 -0.00403
#> 5 2020-01-08 -0.00356 0.0101 0.0153
#> 6 2020-01-09 -0.00258 0.0143 0.00316
#> 7 2020-01-10 -0.00383 -0.00110 -0.00403
#> 8 2020-01-13 0.0158 0.0177 0.00249
#> 9 2020-01-14 0.00262 -0.0128 0.00249
#> 10 2020-01-15 -0.0104 0.00954 -0.00877
#> # ℹ 359 more rows
#>