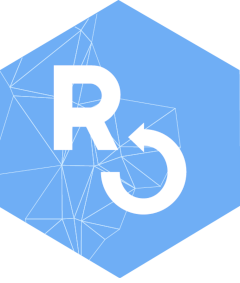
Get the Data Document from the OData Dataset Service.
Source:R/odata_entitylist_data_get.R
odata_entitylist_data_get.Rd
Usage
odata_entitylist_data_get(
pid = get_default_pid(),
did = "",
query = NULL,
url = get_default_url(),
un = get_default_un(),
pw = get_default_pw(),
retries = get_retries(),
odkc_version = get_default_odkc_version(),
orders = get_default_orders(),
tz = get_default_tz()
)
Arguments
- pid
The numeric ID of the project, e.g.: 2.
Default:
get_default_pid
.Set default
pid
throughru_setup(pid="...")
.See
vignette("Setup", package = "ruODK")
.- did
(chr) The name of the Entity List, internally called Dataset. The function will error if this parameter is not given. Default: "".
- query
An optional named list of query parameters, e.g.
list( "$filter" = "__system/createdAt le 2024-11-05", "$orderby" = "__system/creatorId ASC, __system/conflict DESC", "$top" = "100", "$skip" = "3", "$count" = "true" )
No validation is conducted by
ruODK
on the query list prior to passing it to ODK Central. If omitted, no filter query is sent. Note that the behaviour of this parameter differs from the implementation ofodata_submission_get()
in thatquery
here accepts a list of all possible OData query parameters andodata_submission_get()
offers individual function parameters matching supported OData query parameters. Default:NULL
- url
The ODK Central base URL without trailing slash.
Default:
get_default_url
.Set default
url
throughru_setup(url="...")
.See
vignette("Setup", package = "ruODK")
.- un
The ODK Central username (an email address). Default:
get_default_un
. Set defaultun
throughru_setup(un="...")
. Seevignette("Setup", package = "ruODK")
.- pw
The ODK Central password. Default:
get_default_pw
. Set defaultpw
throughru_setup(pw="...")
. Seevignette("Setup", package = "ruODK")
.- retries
The number of attempts to retrieve a web resource.
This parameter is given to
RETRY(times = retries)
.Default: 3.
- odkc_version
The ODK Central version as a semantic version string (year.minor.patch), e.g. "2023.5.1". The version is shown on ODK Central's version page
/version.txt
. Discard the "v".ruODK
uses this parameter to adjust for breaking changes in ODK Central.Default:
get_default_odkc_version
or "2023.5.1" if unset.Set default
get_default_odkc_version
throughru_setup(odkc_version="2023.5.1")
.See
vignette("Setup", package = "ruODK")
.- orders
(vector of character) Orders of datetime elements for lubridate.
Default:
c("YmdHMS", "YmdHMSz", "Ymd HMS", "Ymd HMSz", "Ymd", "ymd")
.- tz
A timezone to convert dates and times to.
Read
vignette("setup", package = "ruODK")
to learn howruODK
's timezone can be set globally or per function.
Value
An S3 class odata_entitylist_data_get
with two list items:
context
The URL for the OData metadata documentvalue
A tibble of EntitySets available in this EntityList, with names cleaned byjanitor::clean_names()
and unnested list columns (__system
).
Details
All the Entities in a Dataset.
The $top
and $skip
querystring parameters, specified by OData, apply limit
and offset operations to the data, respectively.
The $count
parameter, also an OData standard, will annotate the response
data with the total row count, regardless of the scoping requested by $top
and $skip
.
If $top
parameter is provided in the request then the response will include
@odata.nextLink
that you can use as is to fetch the next set of data.
As of ODK Central v2023.4, @odata.nextLink
contains a $skiptoken
(an opaque cursor) to better paginate around deleted Entities.
The $filter
querystring parameter can be used to filter certain data fields
in the system-level schema, but not the Dataset properties.
The operators lt
, le
, eq
, ne
, ge
, gt
, not
, and
, and or
and the built-in functions now
, year
, month
, day
, hour
,
minute
, second
are supported.
The fields you can query against are as follows:
Entity Metadata: OData Field Name
Entity Creator Actor ID: __system/creatorId
Entity Timestamp: __system/createdAt
Entity Update Timestamp: __system/updatedAt
Entity Conflict: __system/conflict
Note that createdAt
and updatedAt
are time components.
This means that any comparisons you make need to account for the full time
of the entity. It might seem like $filter=__system/createdAt le 2020-01-31
would return all results on or before 31 Jan 2020, but in fact only entities
made before midnight of that day would be accepted.
To include all of the month of January, you need to filter by either
$filter=__system/createdAt le 2020-01-31T23:59:59.999Z
or
$filter=__system/createdAt lt 2020-02-01
.
Remember also that you can query by a specific timezone.
Please see the OData documentation on $filter
operations
and functions
for more information.
The $select
query parameter will return just the fields you specify and is
supported on __id
, __system
, __system/creatorId
, __system/createdAt
and __system/updatedAt
, as well as on user defined properties.
The $orderby
query parameter will return Entities sorted by different
fields, which come from the same list used by $filter
, as noted above.
The order can be specified as ASC (ascending) or DESC (descending),
which are case-insensitive. Multiple sort expressions can be used together,
separated by commas,
e.g. $orderby=__system/creatorId ASC, __system/conflict DESC
.
As the vast majority of clients only support the JSON OData format, that is the only format ODK Central offers.
See also
https://docs.getodk.org/central-api-odata-endpoints/#id3
http://docs.oasis-open.org/odata/odata/v4.01/odata-v4.01-part1-protocol.html#_Toc31358948
Other entity-management:
entity_audits()
,
entity_changes()
,
entity_create()
,
entity_delete()
,
entity_detail()
,
entity_list()
,
entity_update()
,
entity_versions()
,
entitylist_detail()
,
entitylist_download()
,
entitylist_list()
,
entitylist_update()
,
odata_entitylist_metadata_get()
,
odata_entitylist_service_get()
Examples
if (FALSE) { # \dontrun{
# See vignette("setup") for setup and authentication options
# ruODK::ru_setup(svc = "....svc", un = "me@email.com", pw = "...")
ds <- entitylist_list(pid = get_default_pid())
ds1 <- odata_entitylist_data_get(pid = get_default_pid(), did = ds$name[1])
ds1
ds1$context
ds1$value
qry <- list(
"$filter" = "__system/createdAt le 2024-11-05",
"$orderby" = "__system/creatorId ASC, __system/conflict DESC",
"$top" = "100",
"$skip" = "3",
"$count" = "true"
)
ds2 <- odata_entitylist_data_get(
pid = get_default_pid(),
did = ds$name[1],
query = qry
)
ds2
} # }