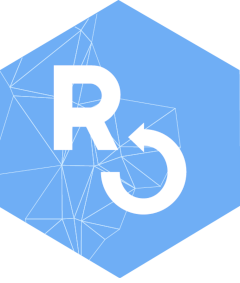
Annotate a dataframe containing a geopoint column with lon, lat, alt.
Source:R/split_geopoint.R
split_geopoint.Rd
Value
The given dataframe with the WKT POINT column colname, plus
three new columns, colname_longitude
, colname_latitude
,
colname_altitude
.
The three new columns are prefixed with the original colname
to
avoid naming conflicts with any other geopoint columns.
Details
This function is used by handle_ru_geopoints
on all geopoint
fields as per form_schema
.
See also
Other utilities:
attachment_get()
,
attachment_link()
,
attachment_url()
,
drop_null_coords()
,
form_schema_parse()
,
get_one_attachment()
,
get_one_submission()
,
get_one_submission_att_list()
,
get_one_submission_audit()
,
handle_ru_attachments()
,
handle_ru_datetimes()
,
handle_ru_geopoints()
,
handle_ru_geoshapes()
,
handle_ru_geotraces()
,
isodt_to_local()
,
odata_submission_rectangle()
,
predict_ruodk_name()
,
prepend_uuid()
,
split_geoshape()
,
split_geotrace()
,
strip_uuid()
,
tidyeval
,
unnest_all()
Examples
if (FALSE) { # \dontrun{
df_wkt <- tibble::tibble(
stuff = c("asd", "sdf", "sdf"),
loc = c(
"POINT (115.99 -32.12 20.01)",
"POINT (116.12 -33.34 15.23)",
"POINT (114.01 -31.56 23.56)"
)
)
df_wkt_split <- df |> split_geopoint("loc", wkt = TRUE)
testthat::expect_equal(
names(df_wkt_split),
c("stuff", "loc", "loc_longitude", "loc_latitude", "loc_altitude")
)
# With package data
data("geo_fs")
data("geo_wkt_raw")
data("geo_gj_raw")
# Find variable names of geopoints
geo_fields <- geo_fs |>
dplyr::filter(type == "geopoint") |>
magrittr::extract2("ruodk_name")
geo_fields[1] # First geotrace in data: point_location_point_gps
# Rectangle but don't parse submission data (GeoJSON and WKT)
geo_gj_rt <- geo_gj_raw |>
odata_submission_rectangle(form_schema = geo_fs)
geo_wkt_rt <- geo_wkt_raw |>
odata_submission_rectangle(form_schema = geo_fs)
# Data with first geopoint split
gj_first_gt <- split_geopoint(geo_gj_rt, geo_fields[1], wkt = FALSE)
gj_first_gt$point_location_point_gps_longitude
wkt_first_gt <- split_geopoint(geo_wkt_rt, geo_fields[1], wkt = TRUE)
wkt_first_gt$point_location_point_gps_longitude
} # }