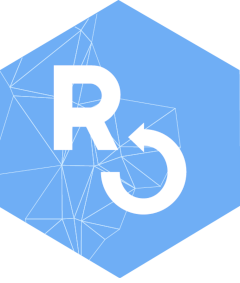
Annotate a dataframe containing a geoshape column with lon, lat, alt of the geotrace's first point.
Source:R/split_geoshape.R
split_geoshape.Rd
Usage
split_geoshape(
data,
colname,
wkt = FALSE,
odkc_version = get_default_odkc_version()
)
Arguments
- data
(dataframe) A dataframe with a geoshape column.
- colname
(chr) The name of the geoshape column. This column will be retained.
- wkt
Whether geofields are GeoJSON (if FALSE) or WKT strings (if TRUE), default: FALSE.
- odkc_version
The ODK Central version as a semantic version string (year.minor.patch), e.g. "2023.5.1". The version is shown on ODK Central's version page
/version.txt
. Discard the "v".ruODK
uses this parameter to adjust for breaking changes in ODK Central.Default:
get_default_odkc_version
or "2023.5.1" if unset.Set default
get_default_odkc_version
throughru_setup(odkc_version="2023.5.1")
.See
vignette("Setup", package = "ruODK")
.
Value
The given dataframe with the geoshape column colname, plus
three new columns, colname_longitude
, colname_latitude
,
colname_altitude
.
The three new columns are prefixed with the original colname
to
avoid naming conflicts with any other geoshape columns.
Details
This function is used by handle_ru_geopoints
on all geopoint
fields as per form_schema
.
See also
Other utilities:
attachment_get()
,
attachment_link()
,
attachment_url()
,
drop_null_coords()
,
form_schema_parse()
,
get_one_attachment()
,
get_one_submission()
,
get_one_submission_att_list()
,
get_one_submission_audit()
,
handle_ru_attachments()
,
handle_ru_datetimes()
,
handle_ru_geopoints()
,
handle_ru_geoshapes()
,
handle_ru_geotraces()
,
isodt_to_local()
,
odata_submission_rectangle()
,
predict_ruodk_name()
,
prepend_uuid()
,
split_geopoint()
,
split_geotrace()
,
strip_uuid()
,
tidyeval
,
unnest_all()
Examples
if (FALSE) { # \dontrun{
library(magrittr)
data("geo_fs")
data("geo_wkt_raw")
data("geo_gj_raw")
# Find variable names of geoshapes
geo_fields <- geo_fs %>%
dplyr::filter(type == "geoshape") %>%
magrittr::extract2("ruodk_name")
geo_fields[1] # First geoshape in data: shape_location_shape_gps
# Rectangle but don't parse submission data (GeoJSON and WKT)
geo_gj_rt <- geo_gj_raw %>%
odata_submission_rectangle(form_schema = geo_fs)
geo_wkt_rt <- geo_wkt_raw %>%
odata_submission_rectangle(form_schema = geo_fs)
# Data with first geoshape split
gj_first_gt <- split_geoshape(geo_gj_rt, geo_fields[1], wkt = FALSE)
cn_gj <- names(gj_first_gt)
testthat::expect_true("shape_location_shape_gps_longitude" %in% cn_gj)
testthat::expect_true("shape_location_shape_gps_latitude" %in% cn_gj)
testthat::expect_true("shape_location_shape_gps_altitude" %in% cn_gj)
testthat::expect_true(
is.numeric(gj_first_gt$shape_location_shape_gps_longitude)
)
testthat::expect_true(
is.numeric(gj_first_gt$shape_location_shape_gps_latitude)
)
testthat::expect_true(
is.numeric(gj_first_gt$shape_location_shape_gps_altitude)
)
wkt_first_gt <- split_geoshape(geo_wkt_rt, geo_fields[1], wkt = TRUE)
cn_wkt <- names(wkt_first_gt)
testthat::expect_true("shape_location_shape_gps_longitude" %in% cn_wkt)
testthat::expect_true("shape_location_shape_gps_latitude" %in% cn_wkt)
testthat::expect_true("shape_location_shape_gps_altitude" %in% cn_wkt)
testthat::expect_true(
is.numeric(wkt_first_gt$shape_location_shape_gps_longitude)
)
testthat::expect_true(
is.numeric(wkt_first_gt$shape_location_shape_gps_latitude)
)
testthat::expect_true(
is.numeric(wkt_first_gt$shape_location_shape_gps_altitude)
)
} # }