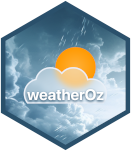
weatherOz for DPIRD
Rodrigo Pires, Anna Hepworth, Rebecca O’Leary and Adam H. Sparks
Source:vignettes/weatherOz_for_DPIRD.Rmd
weatherOz_for_DPIRD.Rmd
About DPIRD Data
From the DPIRD Weather Website’s “About” Page.
The Department of Primary Industries and Regional Development’s (DPIRD) network of automatic weather stations and radars throughout the state provide timely, relevant and local weather data to assist growers and regional communities make more-informed decisions.
The weather station data includes air temperature, humidity, rainfall, wind speed and direction, with most stations also measuring incoming solar radiation to calculate evaporation. This website includes dashboards for each station to visualise this data.
Data from the DPIRD API are licenced under the Creative Commons Attribution 3.0 Licence (CC BY 3.0 AU).
A Note on API Keys
All examples in this vignette assume that you have stored your API key in your .Renviron file. See Chapter 8 in “What They Forgot to Teach You About R” by Bryan et al. for more on storing details in your .Renviron if you are unfamiliar.
Working With DPIRD Data
Three functions are provided to streamline fetching data from the DPIRD Weather 2.0 API endpoints.
-
get_dpird_extremes()
, which returns the recorded extreme values for the given station in the DPIRD weather station network.; -
get_dpird_minute()
, which returns weather data in minute increments for stations in the DPIRD weather station network with only the past two years being available; and -
get_dpird_summaries()
, which returns weather data in 15 and 30 minute, hourly, daily, monthly or yearly summary values for stations in the DPIRD weather station network.
Getting Extreme Weather Values
The get_dpird_extremes()
function fetches and returns
nicely formatted individual extreme weather summaries from the DPIRD
Weather 2.0 API. You must provide a station_code
and
API_key
, the other arguments, values
and
include_closed
are optional.
Available Values for Extreme Weather
- all (which will return all of the following values),
- erosionCondition,
- erosionConditionLast7Days,
- erosionConditionLast7DaysDays,
- erosionConditionLast7DaysMinutes,
- erosionConditionLast14Days,
- erosionConditionLast14DaysDays,
- erosionConditionLast14DaysMinutes,
- erosionConditionMonthToDate,
- erosionConditionMonthToDateDays,
- erosionConditionMonthToDateMinutes,
- erosionConditionMonthToDateStartTime,
- erosionConditionSince12AM,
- erosionConditionSince12AMMinutes,
- erosionConditionSince12AMStartTime,
- erosionConditionYearToDate,
- erosionConditionYearToDateDays,
- erosionConditionYearToDateMinutes,
- erosionConditionYearToDateStartTime,
- frostCondition,
- frostConditionLast7Days,
- frostConditionLast7DaysDays,
- frostConditionLast7DaysMinutes,
- frostConditionLast14Days,
- frostConditionLast14DaysDays,
- frostConditionLast14DaysMinutes,
- frostConditionMonthToDate,
- frostConditionMonthToDateDays,
- frostConditionMonthToDateMinutes,
- frostConditionMonthToDateStartTime,
- frostConditionSince9AM,
- frostConditionSince9AMMinutes,
- frostConditionSince9AMStartTime,
- frostConditionTo9AM,
- frostConditionTo9AMMinutes,
- frostConditionTo9AMStartTime,
- frostConditionYearToDate,
- frostConditionYearToDate,
- frostConditionYearToDateMinutes,
- frostConditionYearToDateStartTime,
- heatCondition,
- heatConditionLast7Days,
- heatConditionLast7DaysDays,
- heatConditionLast7DaysMinutes,
- heatConditionLast14Days,
- heatConditionLast14DaysDays,
- heatConditionLast14DaysMinutes,
- heatConditionMonthToDate,
- heatConditionMonthToDateDays,
- heatConditionMonthToDateMinutes,
- heatConditionMonthToDateStartTime,
- heatConditionSince12AM,
- heatConditionSince12AMMinutes,
- heatConditionSince12AMStartTime,
- heatConditionYearToDate,
- heatConditionYearToDateDays,
- heatConditionYearToDateMinutes, and
- heatConditionYearToDateStartTime
Example 1: Get All Extremes for Northam, WA
In the first example, we illustrate how to fetch all extreme values available for Northam.
library(weatherOz)
(extremes <- get_dpird_extremes(
station_code = "NO"
))
#> Key: <station_code>
#> station_code longitude latitude frost_condition_since9_am_minutes
#> <fctr> <num> <num> <int>
#> 1: NO 116.6942 -31.65161 0
#> frost_condition_since9_am_start_time frost_condition_to9_am_minutes
#> <POSc> <int>
#> 1: <NA> 0
#> frost_condition_to9_am_start_time frost_condition_last7_days_minutes
#> <POSc> <int>
#> 1: <NA> 0
#> frost_condition_last7_days_days frost_condition_last14_days_minutes
#> <int> <int>
#> 1: 0 0
#> frost_condition_last14_days_days frost_condition_month_to_date_minutes
#> <int> <int>
#> 1: 0 0
#> frost_condition_month_to_date_start_time frost_condition_month_to_date_days
#> <POSc> <int>
#> 1: <NA> 0
#> frost_condition_year_to_date_minutes
#> <int>
#> 1: 1125
#> frost_condition_year_to_date_start_time frost_condition_year_to_date_days
#> <POSc> <int>
#> 1: 2024-05-23 05:59:00 9
#> heat_condition_since12_am_minutes heat_condition_since12_am_start_time
#> <int> <POSc>
#> 1: 0 <NA>
#> heat_condition_last7_days_minutes heat_condition_last7_days_days
#> <int> <int>
#> 1: 1255 5
#> heat_condition_last14_days_minutes heat_condition_last14_days_days
#> <int> <int>
#> 1: 1255 5
#> heat_condition_month_to_date_minutes
#> <int>
#> 1: 2075
#> heat_condition_month_to_date_start_time heat_condition_month_to_date_days
#> <POSc> <int>
#> 1: 2024-11-01 09:33:00 6
#> heat_condition_year_to_date_minutes heat_condition_year_to_date_start_time
#> <int> <POSc>
#> 1: 45751 2024-01-01 12:06:00
#> heat_condition_year_to_date_days erosion_condition_since12_am_minutes
#> <int> <int>
#> 1: 108 0
#> erosion_condition_since12_am_start_time
#> <POSc>
#> 1: <NA>
#> erosion_condition_last7_days_minutes erosion_condition_last7_days_days
#> <int> <int>
#> 1: 0 0
#> erosion_condition_last14_days_minutes erosion_condition_last14_days_days
#> <int> <int>
#> 1: 0 0
#> erosion_condition_month_to_date_minutes
#> <int>
#> 1: 0
#> erosion_condition_month_to_date_start_time
#> <POSc>
#> 1: <NA>
#> erosion_condition_month_to_date_days erosion_condition_year_to_date_minutes
#> <int> <int>
#> 1: 0 45
#> erosion_condition_year_to_date_start_time
#> <POSc>
#> 1: 2024-01-13 19:11:00
#> erosion_condition_year_to_date_days
#> <int>
#> 1: 7
Example 2: Get Selected Extremes for Northam, WA
Fetch only soil erosion extreme conditions for Northam, WA. The
documentation for get_dpird_extremes()
contains a full
listing of the values that are available to query from this API
endpoint.
library(weatherOz)
(
extremes <- get_dpird_extremes(
station_code = "NO",
values = "erosionCondition"
)
)
#> Key: <station_code>
#> station_code longitude latitude erosion_condition_since12_am_minutes
#> <fctr> <num> <num> <int>
#> 1: NO 116.6942 -31.65161 0
#> erosion_condition_since12_am_start_time
#> <POSc>
#> 1: <NA>
#> erosion_condition_last7_days_minutes erosion_condition_last7_days_days
#> <int> <int>
#> 1: 0 0
#> erosion_condition_last14_days_minutes erosion_condition_last14_days_days
#> <int> <int>
#> 1: 0 0
#> erosion_condition_month_to_date_minutes
#> <int>
#> 1: 0
#> erosion_condition_month_to_date_start_time
#> <POSc>
#> 1: <NA>
#> erosion_condition_month_to_date_days erosion_condition_year_to_date_minutes
#> <int> <int>
#> 1: 0 45
#> erosion_condition_year_to_date_start_time
#> <POSc>
#> 1: 2024-01-13 19:11:00
#> erosion_condition_year_to_date_days
#> <int>
#> 1: 7
Getting Minute Data
This function fetches nicely formatted minute weather station data
from the DPIRD Weather 2.0 API for a maximum 24-hour period. You must
provide a station_code
and API_key
, the other
arguments, start_date_time
, minutes
and
values
are optional.
Available Values for Minute Data
- all (which will return all of the following values),
- airTemperature,
- dateTime,
- dewPoint,
- rainfall,
- relativeHumidity,
- soilTemperature,
- solarIrradiance,
- wetBulb,
- wind,
- windAvgSpeed,
- windMaxSpeed, and
- windMinSpeed
Example 3: Get All Minute Data for the Past 24 Hours
library(weatherOz)
(
min_dat <- get_dpird_minute(
station_code = "NO"
)
)
#> Key: <station_code>
#> station_code date_time air_temperature relative_humidity
#> <fctr> <POSc> <num> <num>
#> 1: NO 2024-11-16 08:34:00 15.6 40.2
#> 2: NO 2024-11-16 08:35:00 15.6 39.7
#> 3: NO 2024-11-16 08:36:00 15.5 39.7
#> 4: NO 2024-11-16 08:37:00 15.4 40.3
#> 5: NO 2024-11-16 08:38:00 15.3 40.5
#> ---
#> 1428: NO 2024-11-17 08:21:00 16.5 44.4
#> 1429: NO 2024-11-17 08:22:00 16.7 43.7
#> 1430: NO 2024-11-17 08:23:00 16.7 43.8
#> 1431: NO 2024-11-17 08:24:00 16.8 43.3
#> 1432: NO 2024-11-17 08:25:00 16.8 43.1
#> soil_temperature solar_irradiance rainfall dew_point wet_bulb
#> <num> <int> <int> <num> <num>
#> 1: 24.9 762 0 2.1 9.6
#> 2: 25.0 765 0 2.0 9.5
#> 3: 25.0 769 0 1.9 9.4
#> 4: 25.1 772 0 2.0 9.4
#> 5: 25.1 774 0 2.0 9.4
#> ---
#> 1428: 24.1 711 0 4.3 10.8
#> 1429: 24.2 713 0 4.3 10.9
#> 1430: 24.2 716 0 4.3 10.9
#> 1431: 24.2 722 0 4.3 11.0
#> 1432: 24.3 725 0 4.2 10.9
#> wind_height wind_avg_speed wind_avg_direction_compass_point
#> <int> <num> <char>
#> 1: 3 6.70 ESE
#> 2: 3 8.71 SE
#> 3: 3 10.22 SSE
#> 4: 3 11.74 SE
#> 5: 3 9.72 SE
#> ---
#> 1428: 3 8.71 ESE
#> 1429: 3 8.21 ESE
#> 1430: 3 9.72 SE
#> 1431: 3 9.72 SE
#> 1432: 3 7.70 E
#> wind_avg_direction_degrees wind_min_speed wind_max_speed
#> <int> <num> <num>
#> 1: 117 2.664 13.21
#> 2: 132 6.192 11.74
#> 3: 147 5.688 16.24
#> 4: 138 6.192 18.25
#> 5: 145 6.192 13.72
#> ---
#> 1428: 123 5.184 15.23
#> 1429: 112 4.176 13.21
#> 1430: 129 5.688 15.23
#> 1431: 130 7.200 12.71
#> 1432: 82 4.680 15.73
Example 4: Get Specific Time and Date Data for Specific Values
If you wish to supply a specific start date and time and values, you may do so as shown here.
library(weatherOz)
(
min_dat_t_rad_wind <- get_dpird_minute(
station_code = "NO",
start_date_time = "2023-02-01 13:00:00",
minutes = 1440,
values = c("airTemperature",
"solarIrradiance",
"wind")
)
)
#> Key: <station_code>
#> station_code date_time air_temperature solar_irradiance
#> <fctr> <POSc> <num> <int>
#> 1: NO 2023-02-01 13:00:00 29.7 1087
#> 2: NO 2023-02-01 13:00:50 29.4 1086
#> 3: NO 2023-02-01 13:01:40 29.5 1084
#> 4: NO 2023-02-01 13:02:30 29.5 1084
#> 5: NO 2023-02-01 13:03:20 29.6 1084
#> ---
#> 1436: NO 2023-02-02 12:55:00 30.2 1105
#> 1437: NO 2023-02-02 12:55:50 30.1 1104
#> 1438: NO 2023-02-02 12:56:40 30.0 1102
#> 1439: NO 2023-02-02 12:57:30 29.9 1102
#> 1440: NO 2023-02-02 12:58:20 30.1 1104
#> wind_height wind_avg_speed wind_avg_direction_compass_point
#> <int> <num> <char>
#> 1: 3 11.66 SE
#> 2: 3 10.80 SE
#> 3: 3 11.81 SSE
#> 4: 3 12.71 SE
#> 5: 3 12.96 SE
#> ---
#> 1436: 3 9.54 ESE
#> 1437: 3 9.90 ESE
#> 1438: 3 13.03 ESE
#> 1439: 3 10.91 E
#> 1440: 3 10.40 E
#> wind_avg_direction_degrees wind_min_speed wind_max_speed
#> <int> <num> <num>
#> 1: 139 4.176 26.82
#> 2: 136 4.176 19.26
#> 3: 156 6.696 19.26
#> 4: 133 6.696 26.82
#> 5: 139 6.696 19.26
#> ---
#> 1436: 112 4.176 16.74
#> 1437: 120 4.176 19.26
#> 1438: 121 4.176 24.30
#> 1439: 93 6.696 21.78
#> 1440: 97 4.176 16.74
Getting Summary Data
The function, get_dpird_summary()
, fetches nicely
formatted minute weather station data from the DPIRD Weather 2.0 API for
a maximum 24-hour period. You must provide a station_code
and API_key
, the other arguments,
start_date_time
, minutes
and
values
are optional.
Available Values for Summary Data
- all (which will return all of the following values),
- airTemperature,
- airTemperatureAvg,
- airTemperatureMax,
- airTemperatureMaxTime,
- airTemperatureMin,
- airTemperatureMinTime,
- apparentAirTemperature,
- apparentAirTemperatureAvg,
- apparentAirTemperatureMax,
- apparentAirTemperatureMaxTime,
- apparentAirTemperatureMin,
- apparentAirTemperatureMinTime,
- barometricPressure,
- barometricPressureAvg,
- barometricPressureMax,
- barometricPressureMaxTime,
- barometricPressureMin,
- barometricPressureMinTime,
- battery,
- batteryMinVoltage,
- batteryMinVoltageDateTime,
- chillHours,
- deltaT,
- deltaTAvg,
- deltaTMax,
- deltaTMaxTime,
- deltaTMin,
- deltaTMinTime,
- dewPoint,
- dewPointAvg,
- dewPointMax,
- dewPointMaxTime,
- dewPointMin,
- dewPointMinTime,
- erosionCondition,
- erosionConditionMinutes,
- erosionConditionStartTime,
- errors,
- etoShortCrop,
- etoTallCrop,
- evapotranspiration,
- frostCondition,
- frostConditionMinutes,
- frostConditionStartTime,
- heatCondition,
- heatConditionMinutes,
- heatConditionStartTime,
- observations,
- observationsCount,
- observationsPercentage,
- panEvaporation,
- rainfall,
- relativeHumidity,
- relativeHumidityAvg,
- relativeHumidityMax,
- relativeHumidityMaxTime,
- relativeHumidityMin,
- relativeHumidityMinTime,
- richardsonUnits,
- soilTemperature,
- soilTemperatureAvg,
- soilTemperatureMax,
- soilTemperatureMaxTime,
- soilTemperatureMin,
- soilTemperatureMinTime,
- solarExposure,
- wetBulb,
- wetBulbAvg,
- wetBulbMax,
- wetBulbMaxTime,
- wetBulbMin,
- wetBulbMinTime,
- wind,
- windAvgSpeed, and
- windMaxSpeed
What You Get Back
This function returns a data.table
with
station_code
and the date interval queried together with
the requested weather variables in alphabetical order. Please note this
function converts date-time columns from Coordinated Universal Time
‘UTC’} to Australian Western Standard Time ‘AWST’. The first ten columns
will always be:
-
station_code
, -
station_name
, -
longitude
, -
latitude
, -
year
, -
month
, -
day
, -
hour
, -
minute
, and ifmonth
or finer is present, -
date
(a combination of year, month, day, hour, minute as appropriate)
Example 5: Get Annual Rainfall Since 2017
Use the default value for end date (current system date) to get annual rainfall since 2017 until current year for Capel.
library(weatherOz)
(
annual_rain <- get_dpird_summaries(
station_code = "CL001",
start_date = "20170101",
interval = "yearly",
values = "rainfall"
)
)
#> Key: <station_code>
#> station_code station_name longitude latitude year rainfall
#> <fctr> <char> <num> <num> <int> <num>
#> 1: CL001 Capel 115.6376 -33.61576 2017 711.4
#> 2: CL001 Capel 115.6376 -33.61576 2018 822.0
#> 3: CL001 Capel 115.6376 -33.61576 2019 660.6
#> 4: CL001 Capel 115.6376 -33.61576 2020 862.4
#> 5: CL001 Capel 115.6376 -33.61576 2021 928.0
#> 6: CL001 Capel 115.6376 -33.61576 2022 670.4
#> 7: CL001 Capel 115.6376 -33.61576 2023 570.0
#> 8: CL001 Capel 115.6376 -33.61576 2024 760.2
Example 6: Get Monthly Rainfall Since 2017
Use the default value for end date (current system date) to get monthly rainfall since 2017 until current year for Capel.
library(weatherOz)
(
monthly_rain <- get_dpird_summaries(
station_code = "CL001",
start_date = "20170101",
interval = "monthly",
values = "rainfall"
)
)
#> Key: <station_code>
#> station_code station_name longitude latitude year month date
#> <fctr> <char> <num> <num> <int> <int> <Date>
#> 1: CL001 Capel 115.6376 -33.61576 2017 1 2017-01-01
#> 2: CL001 Capel 115.6376 -33.61576 2017 2 2017-02-01
#> 3: CL001 Capel 115.6376 -33.61576 2017 3 2017-03-01
#> 4: CL001 Capel 115.6376 -33.61576 2017 4 2017-04-01
#> 5: CL001 Capel 115.6376 -33.61576 2017 5 2017-05-01
#> 6: CL001 Capel 115.6376 -33.61576 2017 6 2017-06-01
#> 7: CL001 Capel 115.6376 -33.61576 2017 7 2017-07-01
#> 8: CL001 Capel 115.6376 -33.61576 2017 8 2017-08-01
#> 9: CL001 Capel 115.6376 -33.61576 2017 9 2017-09-01
#> 10: CL001 Capel 115.6376 -33.61576 2017 10 2017-10-01
#> 11: CL001 Capel 115.6376 -33.61576 2017 11 2017-11-01
#> 12: CL001 Capel 115.6376 -33.61576 2017 12 2017-12-01
#> 13: CL001 Capel 115.6376 -33.61576 2018 1 2018-01-01
#> 14: CL001 Capel 115.6376 -33.61576 2018 2 2018-02-01
#> 15: CL001 Capel 115.6376 -33.61576 2018 3 2018-03-01
#> 16: CL001 Capel 115.6376 -33.61576 2018 4 2018-04-01
#> 17: CL001 Capel 115.6376 -33.61576 2018 5 2018-05-01
#> 18: CL001 Capel 115.6376 -33.61576 2018 6 2018-06-01
#> 19: CL001 Capel 115.6376 -33.61576 2018 7 2018-07-01
#> 20: CL001 Capel 115.6376 -33.61576 2018 8 2018-08-01
#> 21: CL001 Capel 115.6376 -33.61576 2018 9 2018-09-01
#> 22: CL001 Capel 115.6376 -33.61576 2018 10 2018-10-01
#> 23: CL001 Capel 115.6376 -33.61576 2018 11 2018-11-01
#> 24: CL001 Capel 115.6376 -33.61576 2018 12 2018-12-01
#> 25: CL001 Capel 115.6376 -33.61576 2019 1 2019-01-01
#> 26: CL001 Capel 115.6376 -33.61576 2019 2 2019-02-01
#> 27: CL001 Capel 115.6376 -33.61576 2019 3 2019-03-01
#> 28: CL001 Capel 115.6376 -33.61576 2019 4 2019-04-01
#> 29: CL001 Capel 115.6376 -33.61576 2019 5 2019-05-01
#> 30: CL001 Capel 115.6376 -33.61576 2019 6 2019-06-01
#> 31: CL001 Capel 115.6376 -33.61576 2019 7 2019-07-01
#> 32: CL001 Capel 115.6376 -33.61576 2019 8 2019-08-01
#> 33: CL001 Capel 115.6376 -33.61576 2019 9 2019-09-01
#> 34: CL001 Capel 115.6376 -33.61576 2019 10 2019-10-01
#> 35: CL001 Capel 115.6376 -33.61576 2019 11 2019-11-01
#> 36: CL001 Capel 115.6376 -33.61576 2019 12 2019-12-01
#> 37: CL001 Capel 115.6376 -33.61576 2020 1 2020-01-01
#> 38: CL001 Capel 115.6376 -33.61576 2020 2 2020-02-01
#> 39: CL001 Capel 115.6376 -33.61576 2020 3 2020-03-01
#> 40: CL001 Capel 115.6376 -33.61576 2020 4 2020-04-01
#> 41: CL001 Capel 115.6376 -33.61576 2020 5 2020-05-01
#> 42: CL001 Capel 115.6376 -33.61576 2020 6 2020-06-01
#> 43: CL001 Capel 115.6376 -33.61576 2020 7 2020-07-01
#> 44: CL001 Capel 115.6376 -33.61576 2020 8 2020-08-01
#> 45: CL001 Capel 115.6376 -33.61576 2020 9 2020-09-01
#> 46: CL001 Capel 115.6376 -33.61576 2020 10 2020-10-01
#> 47: CL001 Capel 115.6376 -33.61576 2020 11 2020-11-01
#> 48: CL001 Capel 115.6376 -33.61576 2020 12 2020-12-01
#> 49: CL001 Capel 115.6376 -33.61576 2021 1 2021-01-01
#> 50: CL001 Capel 115.6376 -33.61576 2021 2 2021-02-01
#> 51: CL001 Capel 115.6376 -33.61576 2021 3 2021-03-01
#> 52: CL001 Capel 115.6376 -33.61576 2021 4 2021-04-01
#> 53: CL001 Capel 115.6376 -33.61576 2021 5 2021-05-01
#> 54: CL001 Capel 115.6376 -33.61576 2021 6 2021-06-01
#> 55: CL001 Capel 115.6376 -33.61576 2021 7 2021-07-01
#> 56: CL001 Capel 115.6376 -33.61576 2021 8 2021-08-01
#> 57: CL001 Capel 115.6376 -33.61576 2021 9 2021-09-01
#> 58: CL001 Capel 115.6376 -33.61576 2021 10 2021-10-01
#> 59: CL001 Capel 115.6376 -33.61576 2021 11 2021-11-01
#> 60: CL001 Capel 115.6376 -33.61576 2021 12 2021-12-01
#> 61: CL001 Capel 115.6376 -33.61576 2022 1 2022-01-01
#> 62: CL001 Capel 115.6376 -33.61576 2022 2 2022-02-01
#> 63: CL001 Capel 115.6376 -33.61576 2022 3 2022-03-01
#> 64: CL001 Capel 115.6376 -33.61576 2022 4 2022-04-01
#> 65: CL001 Capel 115.6376 -33.61576 2022 5 2022-05-01
#> 66: CL001 Capel 115.6376 -33.61576 2022 6 2022-06-01
#> 67: CL001 Capel 115.6376 -33.61576 2022 7 2022-07-01
#> 68: CL001 Capel 115.6376 -33.61576 2022 8 2022-08-01
#> 69: CL001 Capel 115.6376 -33.61576 2022 9 2022-09-01
#> 70: CL001 Capel 115.6376 -33.61576 2022 10 2022-10-01
#> 71: CL001 Capel 115.6376 -33.61576 2022 11 2022-11-01
#> 72: CL001 Capel 115.6376 -33.61576 2022 12 2022-12-01
#> 73: CL001 Capel 115.6376 -33.61576 2023 1 2023-01-01
#> 74: CL001 Capel 115.6376 -33.61576 2023 2 2023-02-01
#> 75: CL001 Capel 115.6376 -33.61576 2023 3 2023-03-01
#> 76: CL001 Capel 115.6376 -33.61576 2023 4 2023-04-01
#> 77: CL001 Capel 115.6376 -33.61576 2023 5 2023-05-01
#> 78: CL001 Capel 115.6376 -33.61576 2023 6 2023-06-01
#> 79: CL001 Capel 115.6376 -33.61576 2023 7 2023-07-01
#> 80: CL001 Capel 115.6376 -33.61576 2023 8 2023-08-01
#> 81: CL001 Capel 115.6376 -33.61576 2023 9 2023-09-01
#> 82: CL001 Capel 115.6376 -33.61576 2023 10 2023-10-01
#> 83: CL001 Capel 115.6376 -33.61576 2023 11 2023-11-01
#> 84: CL001 Capel 115.6376 -33.61576 2023 12 2023-12-01
#> 85: CL001 Capel 115.6376 -33.61576 2024 1 2024-01-01
#> 86: CL001 Capel 115.6376 -33.61576 2024 2 2024-02-01
#> 87: CL001 Capel 115.6376 -33.61576 2024 3 2024-03-01
#> 88: CL001 Capel 115.6376 -33.61576 2024 4 2024-04-01
#> 89: CL001 Capel 115.6376 -33.61576 2024 5 2024-05-01
#> 90: CL001 Capel 115.6376 -33.61576 2024 6 2024-06-01
#> 91: CL001 Capel 115.6376 -33.61576 2024 7 2024-07-01
#> 92: CL001 Capel 115.6376 -33.61576 2024 8 2024-08-01
#> 93: CL001 Capel 115.6376 -33.61576 2024 9 2024-09-01
#> 94: CL001 Capel 115.6376 -33.61576 2024 10 2024-10-01
#> 95: CL001 Capel 115.6376 -33.61576 2024 11 2024-11-01
#> station_code station_name longitude latitude year month date
#> rainfall
#> <num>
#> 1: 0.0
#> 2: 0.0
#> 3: 59.6
#> 4: 0.0
#> 5: 84.0
#> 6: 57.8
#> 7: 194.4
#> 8: 175.8
#> 9: 49.8
#> 10: 34.0
#> 11: 14.2
#> 12: 41.8
#> 13: 14.4
#> 14: 0.2
#> 15: 15.4
#> 16: 42.8
#> 17: 115.2
#> 18: 168.8
#> 19: 214.6
#> 20: 156.8
#> 21: 29.6
#> 22: 45.8
#> 23: 11.2
#> 24: 7.2
#> 25: 6.0
#> 26: 0.0
#> 27: 29.4
#> 28: 33.0
#> 29: 27.0
#> 30: 239.6
#> 31: 85.4
#> 32: 117.8
#> 33: 45.8
#> 34: 67.0
#> 35: 7.8
#> 36: 1.8
#> 37: 2.0
#> 38: 31.8
#> 39: 40.8
#> 40: 41.6
#> 41: 156.2
#> 42: 132.4
#> 43: 125.8
#> 44: 96.0
#> 45: 110.0
#> 46: 32.6
#> 47: 88.0
#> 48: 5.2
#> 49: 0.0
#> 50: 76.2
#> 51: 27.4
#> 52: 92.0
#> 53: 160.6
#> 54: 81.2
#> 55: 187.6
#> 56: 90.4
#> 57: 93.4
#> 58: 109.4
#> 59: 5.4
#> 60: 4.4
#> 61: 0.0
#> 62: 4.4
#> 63: 4.6
#> 64: 44.0
#> 65: 105.6
#> 66: 103.8
#> 67: 169.0
#> 68: 121.4
#> 69: 69.4
#> 70: 29.0
#> 71: 18.0
#> 72: 1.2
#> 73: 0.8
#> 74: 0.0
#> 75: 27.8
#> 76: 81.2
#> 77: 31.8
#> 78: 130.0
#> 79: 123.2
#> 80: 74.8
#> 81: 80.2
#> 82: 17.2
#> 83: 2.4
#> 84: 0.6
#> 85: 1.4
#> 86: 0.6
#> 87: 0.2
#> 88: 0.0
#> 89: 60.6
#> 90: 202.2
#> 91: 182.8
#> 92: 226.8
#> 93: 34.0
#> 94: 48.4
#> 95: 3.2
#> rainfall
Example 7: Get Daily Rainfall and Wind From Beginning of 2017 to End of 2018
Use the default value for end date (current system date) to get daily rainfall and wind records from 2017-01-01 to 2018-12-31 for Binnu. Note that the Binnu station has two wind heights, 3m and 10m.
library(weatherOz)
(
daily_wind_rain <- get_dpird_summaries(
station_code = "BI",
start_date = "20170101",
end_date = "2018-12-31",
interval = "daily",
values = c("rainfall",
"wind")
)
)
#> Key: <station_code>
#> station_code station_name longitude latitude year month day
#> <fctr> <char> <num> <num> <int> <int> <int>
#> 1: BI Binnu 114.6958 -28.051 2017 1 1
#> 2: BI Binnu 114.6958 -28.051 2017 1 1
#> 3: BI Binnu 114.6958 -28.051 2017 1 2
#> 4: BI Binnu 114.6958 -28.051 2017 1 2
#> 5: BI Binnu 114.6958 -28.051 2017 1 3
#> ---
#> 1456: BI Binnu 114.6958 -28.051 2018 12 29
#> 1457: BI Binnu 114.6958 -28.051 2018 12 30
#> 1458: BI Binnu 114.6958 -28.051 2018 12 30
#> 1459: BI Binnu 114.6958 -28.051 2018 12 31
#> 1460: BI Binnu 114.6958 -28.051 2018 12 31
#> date rainfall wind_avg_speed wind_height
#> <Date> <num> <num> <int>
#> 1: 2017-01-01 0 17.61 3
#> 2: 2017-01-01 0 20.25 3
#> 3: 2017-01-02 0 23.30 10
#> 4: 2017-01-02 0 25.59 10
#> 5: 2017-01-03 0 16.89 3
#> ---
#> 1456: 2018-12-29 0 26.38 10
#> 1457: 2018-12-30 0 17.37 3
#> 1458: 2018-12-30 0 19.32 3
#> 1459: 2018-12-31 0 22.30 10
#> 1460: 2018-12-31 0 24.58 10
#> wind_max_direction_compass_point wind_max_direction_degrees
#> <char> <int>
#> 1: SW 220
#> 2: SSW 200
#> 3: ESE 104
#> 4: SSW 208
#> 5: SSW 198
#> ---
#> 1456: SSW 193
#> 1457: SSW 201
#> 1458: SSW 196
#> 1459: SSW 198
#> 1460: SSW 197
#> wind_max_speed wind_max_time
#> <num> <POSc>
#> 1: 42.73 2017-01-01 17:56:00
#> 2: 42.73 2018-01-01 17:25:00
#> 3: 46.30 2017-01-01 08:08:00
#> 4: 44.64 2018-01-01 17:42:00
#> 5: 47.77 2017-01-02 17:02:00
#> ---
#> 1456: 50.15 2018-12-30 16:13:00
#> 1457: 47.77 2017-12-31 16:41:00
#> 1458: 41.90 2018-12-31 17:13:00
#> 1459: 51.73 2017-12-31 16:19:00
#> 1460: 44.78 2018-12-31 18:12:00
Example 8: Get Hourly Rainfall and Wind From Beginning of 2022 to Current
Use the default value for end date (current system date) to get hourly rainfall and wind records from 2022-01-01 to Current Date for Binnu. Note that the Binnu station has two wind heights, 3m and 10m.
library(weatherOz)
(
hourly_wind_rain <- get_dpird_summaries(
station_code = "BI",
start_date = "20220101",
interval = "hourly",
values = c("rainfall",
"wind")
)
)
#> Key: <station_code>
#> station_code station_name longitude latitude year month day hour
#> <fctr> <char> <num> <num> <int> <int> <int> <int>
#> 1: BI Binnu 114.6958 -28.051 2022 1 1 0
#> 2: BI Binnu 114.6958 -28.051 2022 1 1 0
#> 3: BI Binnu 114.6958 -28.051 2022 1 1 1
#> 4: BI Binnu 114.6958 -28.051 2022 1 1 1
#> 5: BI Binnu 114.6958 -28.051 2022 1 1 2
#> ---
#> 50446: BI Binnu 114.6958 -28.051 2024 11 16 22
#> 50447: BI Binnu 114.6958 -28.051 2024 11 16 23
#> 50448: BI Binnu 114.6958 -28.051 2024 11 16 23
#> 50449: BI Binnu 114.6958 -28.051 2024 11 17 0
#> 50450: BI Binnu 114.6958 -28.051 2024 11 17 0
#> date rainfall wind_avg_direction_compass_point
#> <POSc> <num> <char>
#> 1: 2022-01-01 00:00:00 0 S
#> 2: 2022-01-01 00:00:00 0 NW
#> 3: 2022-01-01 01:00:00 0 S
#> 4: 2022-01-01 01:00:00 0 WNW
#> 5: 2022-01-01 02:00:00 0 S
#> ---
#> 50446: 2024-11-16 22:00:00 0 S
#> 50447: 2024-11-16 23:00:00 0 NW
#> 50448: 2024-11-16 23:00:00 0 S
#> 50449: 2024-11-17 00:00:00 0 NNW
#> 50450: 2024-11-17 00:00:00 0 SSE
#> wind_avg_direction_degrees wind_avg_speed wind_height
#> <int> <num> <int>
#> 1: 190 16.66 3
#> 2: 325 10.84 10
#> 3: 189 22.23 10
#> 4: 299 12.76 3
#> 5: 190 22.65 3
#> ---
#> 50446: 174 29.25 10
#> 50447: 323 8.13 10
#> 50448: 170 20.24 3
#> 50449: 327 8.86 3
#> 50450: 164 30.16 10
#> wind_max_direction_compass_point wind_max_direction_degrees
#> <char> <int>
#> 1: S 184
#> 2: NW 310
#> 3: S 180
#> 4: WNW 289
#> 5: SSW 192
#> ---
#> 50446: S 173
#> 50447: NW 321
#> 50448: SSE 167
#> 50449: NNW 330
#> 50450: SSE 164
#> wind_max_speed wind_max_time
#> <num> <POSc>
#> 1: 30.13 2021-12-31 23:58:00
#> 2: 18.04 2023-06-10 11:20:00
#> 3: 32.08 2021-12-31 23:58:00
#> 4: 25.13 2023-06-10 12:41:00
#> 5: 32.65 2022-01-01 00:29:00
#> ---
#> 50446: 40.72 2024-11-16 22:41:00
#> 50447: 14.40 2023-06-10 10:59:00
#> 50448: 32.76 2024-11-16 23:44:00
#> 50449: 18.40 2023-06-10 11:37:00
#> 50450: 42.19 2024-11-16 23:19:00
Getting APSIM-ready Data
For work with APSIM, you can use get_dpird_apsim()
to
get an object of DPIRD weather data in your R session that’s ready for
saving using write_apsim_met()
, which is re-exported from
the CRAN package [apsimx] for your convenience. This function only needs
the station_code
, start_date
,
end_date
and your api_key
values to return the
necessary values.
What You Get Back
An object of {apsimx} ‘met’ class, compatible with a
data.frame
, that has daily data that include year, day,
radiation, max temperature, min temperature, rainfall, relative
humidity, evaporation and windspeed.
Example 9: Get APSIM Formatted Data for Binnu From 2022-04-01 to 2022-11-01
library(weatherOz)
(
binnu <- get_dpird_apsim(
station_code = "BI",
start_date = "20220101",
end_date = "20221231"
)
)
#> weather.met.met
#> site = Binnu
#> latitude = -28.051
#> longitude = 114.69575
#> tav = 20.5987671232877 (oC) ! calculated annual average ambient temperature 2024-11-17 08:34:35.872418
#> amp = 15.3 !calculated with the apsimx R package: 2024-11-17 08:34:35.874992
#> year day radn maxt mint rain evap rh windspeed
#> () () (MJ/m2/day) (oC) (oC) (mm) (mm) (%) (m/s)
#> year day radn maxt mint rain evap rh windspeed
#> 1 2022 1 33137.3 35.3 18.5 10.6 0 63.9 20.52
#> 2 2022 2 33419.1 38.3 15.2 11.1 0 54.6 15.03
#> 3 2022 3 33467.9 34.8 17.1 11.0 0 65.6 18.62
#> 4 2022 4 32784.2 39.9 17.6 11.2 0 64.2 18.44
#> 5 2022 5 32981.1 45.7 19.1 14.4 0 37.1 18.09
#> 6 2022 6 33182.6 39.1 18.3 11.3 0 60.5 18.37
#> Warning in check_apsim_met(x): Radiation is greater than 40 (MJ/m2/day)
#> weather.met.met
#> site = Binnu
#> latitude = -28.051
#> longitude = 114.69575
#> tav = 20.5987671232877 (oC) ! calculated annual average ambient temperature 2024-11-17 08:34:35.872418
#> amp = 15.3 !calculated with the apsimx R package: 2024-11-17 08:34:35.874992
#> year day radn maxt mint rain evap rh windspeed
#> () () (MJ/m2/day) (oC) (oC) (mm) (mm) (%) (m/s)
#> year day radn maxt mint rain evap rh windspeed
#> 1 2022 1 33137.3 35.3 18.5 10.6 0 63.9 20.52
#> 2 2022 2 33419.1 38.3 15.2 11.1 0 54.6 15.03
#> 3 2022 3 33467.9 34.8 17.1 11.0 0 65.6 18.62
#> 4 2022 4 32784.2 39.9 17.6 11.2 0 64.2 18.44
#> 5 2022 5 32981.1 45.7 19.1 14.4 0 37.1 18.09
#> 6 2022 6 33182.6 39.1 18.3 11.3 0 60.5 18.37
Working With DPIRD Metadata
Three functions are provided to assist in fetching metadata about the stations.
-
find_nearby_stations()
, which returns adata.table
with the nearest weather stations to a given geographic point or known station in either the DPIRD or BOM (from SILO) networks. -
find_stations_in()
, which returns adata.table
with the weather stations falling within a given geographic area in either the DPIRD or BOM (from SILO) networks. -
get_dpird_availability()
, which returns adata.table
with the availability for weather stations in the DPIRD network providing the up time and data availability for a given period of time. -
get_stations_metadata()
, which returns adata.table
with the latest and most up-to-date information available from the Weather 2.0 API on the stations’ geographic locations, hardware details, e.g., wind mast height, and recording capabilities.
Example 10: Finding Stations Nearby a Known Station
Query WA only stations and return DPIRD’s stations nearest to the Northam, WA station, “NO”, returning stations with 50 km of this station.
library(weatherOz)
(
wa_stn <- find_nearby_stations(
station_code = "NO",
distance_km = 50,
which_api = "dpird"
)
)
#> station_code station_name longitude latitude state elev_m
#> <fctr> <char> <num> <num> <char> <int>
#> 1: NO Northam 116.6942 -31.65161 WA 163
#> 2: MK Muresk 116.6913 -31.72772 WA 251
#> 3: YE001 York East 116.9211 -31.83588 WA 229
#> 4: BTSB DFES-B Talbot West 116.6898 -31.96060 WA 350
#> owner
#> <char>
#> 1: WA Department of Primary Industries and Regional Development (DPIRD)
#> 2: WA Department of Primary Industries and Regional Development (DPIRD)
#> 3: WA Department of Primary Industries and Regional Development (DPIRD)
#> 4: WA Department of Fire and Emergency Services (DFES)
#> distance_km
#> <num>
#> 1: 0.00
#> 2: 7.62
#> 3: 29.23
#> 4: 30.90
Example 11: Finding Stations Nearby a Given Longitude and Latitude
Using the longitude and latitude for Northam, WA, find all DPIRD stations within a 50km radius of this geographic point.
library(weatherOz)
(
wa_stn_lonlat <- find_nearby_stations(
longitude = 116.6620,
latitude = -31.6540,
distance_km = 50,
which_api = "dpird"
)
)
#> station_code station_name longitude latitude state elev_m
#> <fctr> <char> <num> <num> <char> <int>
#> 1: NO Northam 116.6942 -31.65161 WA 163
#> 2: MK Muresk 116.6913 -31.72772 WA 251
#> 3: BTSB DFES-B Talbot West 116.6898 -31.96060 WA 350
#> 4: YE001 York East 116.9211 -31.83588 WA 229
#> owner
#> <char>
#> 1: WA Department of Primary Industries and Regional Development (DPIRD)
#> 2: WA Department of Primary Industries and Regional Development (DPIRD)
#> 3: WA Department of Fire and Emergency Services (DFES)
#> 4: WA Department of Primary Industries and Regional Development (DPIRD)
#> distance_km
#> <num>
#> 1: 3.23
#> 2: 7.93
#> 3: 30.79
#> 4: 31.65
Example 12: Finding Stations in Both the DPIRD and SILO Data Sets
Query stations nearest DPIRD’s Northam, WA station, “NO” and return both DPIRD and SILO/BOM stations within 50 km of this station.
library(weatherOz)
(
wa_stn_all <- find_nearby_stations(
station_code = "NO",
distance_km = 50,
which_api = "all"
)
)
#> station_code station_name longitude latitude state elev_m
#> <fctr> <char> <num> <num> <char> <num>
#> 1: NO Northam 116.6942 -31.65161 WA 163
#> 2: 010111 Northam 116.6586 -31.65080 WA 170
#> 3: MK Muresk 116.6913 -31.72772 WA 251
#> 4: 010150 Grass Valley 116.7969 -31.63580 WA 200
#> 5: 010152 Muresk Institute 116.6833 -31.75000 WA 166
#> 6: 010115 Quellington 116.8647 -31.77140 WA 220
#> 7: 010125 Toodyay 116.4703 -31.55170 WA 140
#> 8: 010244 Bakers Hill 116.4561 -31.74690 WA 330
#> 9: 010311 York 116.7650 -31.89970 WA 179
#> 10: 010023 Warradong Farm 116.9411 -31.50030 WA 240
#> 11: YE001 York East 116.9211 -31.83588 WA 229
#> 12: 010091 Meckering 117.0081 -31.63220 WA 195
#> 13: BTSB DFES-B Talbot West 116.6898 -31.96060 WA 350
#> 14: 010138 Wooroloo 116.3413 -31.81500 WA 277
#> 15: 010058 Goomalling 116.8269 -31.29940 WA 239
#> 16: 010134 Wattening 116.5150 -31.31190 WA 240
#> 17: 010165 Green Hills 116.9839 -31.94080 WA 244
#> 18: 010163 Jaroma 117.1433 -31.77060 WA 265
#> 19: 010009 Bolgart 116.5092 -31.27440 WA 240
#> 20: 010160 Quella Park 117.1194 -31.45330 WA 265
#> 21: 009007 Chidlow 116.2658 -31.86220 WA 300
#> 22: 010120 Doodenanning 117.0986 -31.90920 WA 290
#> 23: 009066 Gidgegannup 116.1976 -31.79060 WA 290
#> station_code station_name longitude latitude state elev_m
#> owner
#> <char>
#> 1: WA Department of Primary Industries and Regional Development (DPIRD)
#> 2: BOM
#> 3: WA Department of Primary Industries and Regional Development (DPIRD)
#> 4: BOM
#> 5: BOM
#> 6: BOM
#> 7: BOM
#> 8: BOM
#> 9: BOM
#> 10: BOM
#> 11: WA Department of Primary Industries and Regional Development (DPIRD)
#> 12: BOM
#> 13: WA Department of Fire and Emergency Services (DFES)
#> 14: BOM
#> 15: BOM
#> 16: BOM
#> 17: BOM
#> 18: BOM
#> 19: BOM
#> 20: BOM
#> 21: BOM
#> 22: BOM
#> 23: BOM
#> owner
#> distance_km
#> <num>
#> 1: 0.000000
#> 2: 3.369959
#> 3: 7.620000
#> 4: 9.878912
#> 5: 10.986728
#> 6: 20.914807
#> 7: 23.934879
#> 8: 24.889495
#> 9: 28.381350
#> 10: 28.808439
#> 11: 29.230000
#> 12: 29.790490
#> 13: 30.900000
#> 14: 37.993263
#> 15: 41.128932
#> 16: 41.412805
#> 17: 42.226585
#> 18: 44.489821
#> 19: 45.457855
#> 20: 45.923973
#> 21: 46.778842
#> 22: 47.759247
#> 23: 49.440793
#> distance_km
Example 13: Finding Stations in the Southwest Agriculture Region of Western Australia
Using find_stations_in()
is different than
find_nearby_stations()
as it finds any stations that fall
within a boundary that you provide rather than using a single point to
search from. For detailed examples using named places or bounding boxes,
see the “weatherOz for SILO” vignette.
The {sf} object, south_west_agricultural_region
, is
provided with {weatherOz} under the CC BY 4.0
Licence from the Department of Primary Industries and Regional
Development (DPIRD), so we can extract stations within this area of
Western Australia.
First, we can plot the south_west_agricultural_region
to
see what it looks like.
library(weatherOz)
library(ggplot2)
library(ggthemes)
library(sf)
ggplot(south_west_agricultural_region) +
geom_sf() +
theme_map()
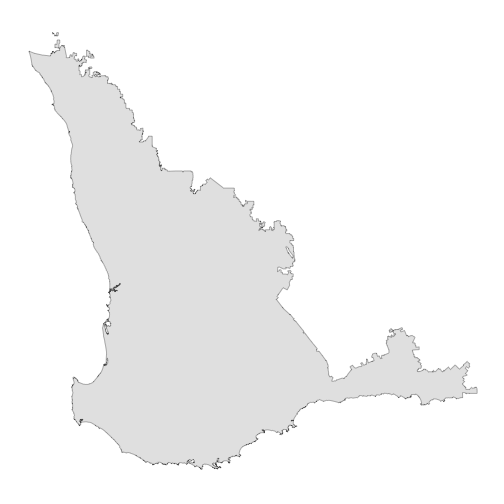
plot of chunk view_sw_ag_region
Now we can use that to find stations that fall only within that part of Western Australia. We’ll use the coordinate reference system (CRS) provided by this {sf} object and find all stations, including those that have closed.
sw_wa <- find_stations_in(
x = south_west_agricultural_region,
include_closed = TRUE,
crs = sf::st_crs(south_west_agricultural_region)
)
sw_wa
#> station_code station_name start end latitude
#> <fctr> <char> <Date> <Date> <num>
#> 1: 009804 Adina 1969-01-01 2024-11-17 -33.88110
#> 2: 008000 Ajana 1917-01-01 2024-11-17 -27.96070
#> 3: 009500 Albany 1877-01-01 2024-11-17 -35.02890
#> 4: 009741 Albany Airport Comparison 1942-01-01 2014-01-01 -34.94140
#> 5: 010501 Aldersyde Post Office 1909-01-01 1976-01-01 -32.36670
#> ---
#> 1034: 008146 Ytiniche 1913-01-01 2024-11-17 -30.07060
#> 1035: 008147 Yuna 1909-01-01 2024-11-17 -28.32500
#> 1036: YU001 Yuna 2012-06-21 2024-11-17 -28.33763
#> 1037: YU002 Yuna NE 2016-03-24 2024-11-17 -28.20032
#> 1038: YU003 Yuna North 2018-08-08 2024-11-17 -28.12086
#> longitude state elev_m
#> <num> <char> <num>
#> 1: 122.2167 WA 60
#> 2: 114.6336 WA 210
#> 3: 117.8808 WA 3
#> 4: 117.8022 WA 68
#> 5: 117.2833 WA NA
#> ---
#> 1034: 116.2092 WA 300
#> 1035: 114.9589 WA 270
#> 1036: 114.9898 WA 329
#> 1037: 115.2616 WA 267
#> 1038: 114.9626 WA 264
#> source
#> <char>
#> 1: Bureau of Meteorology (BOM)
#> 2: Bureau of Meteorology (BOM)
#> 3: Bureau of Meteorology (BOM)
#> 4: Bureau of Meteorology (BOM)
#> 5: Bureau of Meteorology (BOM)
#> ---
#> 1034: Bureau of Meteorology (BOM)
#> 1035: Bureau of Meteorology (BOM)
#> 1036: WA Department of Primary Industries and Regional Development (DPIRD)
#> 1037: WA Department of Primary Industries and Regional Development (DPIRD)
#> 1038: WA Department of Primary Industries and Regional Development (DPIRD)
#> status wmo
#> <char> <num>
#> 1: open NA
#> 2: open NA
#> 3: open 94801
#> 4: closed 95802
#> 5: closed NA
#> ---
#> 1034: open NA
#> 1035: open NA
#> 1036: open NA
#> 1037: open NA
#> 1038: open NA
We need to convert the sw_wa
object from a
data.table
to an sf
object and transform it to
use the same CRS as the south_west_agricultural_region
object to map the results.
sw_wa <- st_as_sf(
x = sw_wa,
coords = c("longitude", "latitude"),
crs = "EPSG:4326"
)
sw_wa <- st_transform(x = sw_wa, crs = st_crs(south_west_agricultural_region))
Now we can use {ggplot2} to plot the stations indicating whether they are still open or they are closed.
ggplot(south_west_agricultural_region) +
geom_sf(fill = "white") +
geom_sf(data = sw_wa,
alpha = 0.65,
size = 2,
aes(colour = status)) +
theme_map()
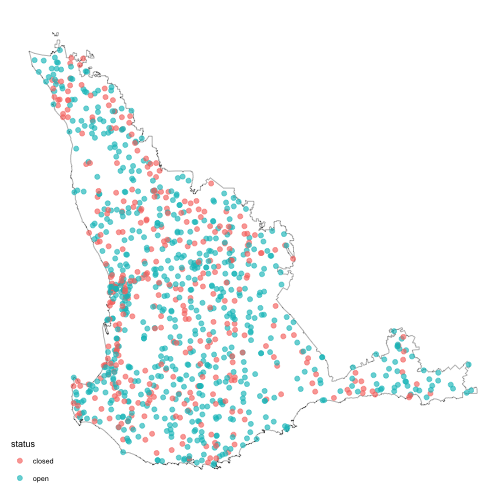
plot of chunk plot_sw_land_div_map
Example 14: Checking Station Availability for Current Year
Check the availability of the Westonia station since the start of the
current year using the default functionality with no
start_date
or end_date
.
library(weatherOz)
(WS001 <- get_dpird_availability(
station_code = "WS001"
))
#> Key: <station_code>
#> station_code station_name to9_am since9_am since12_am current_hour
#> <fctr> <char> <int> <int> <int> <int>
#> 1: WS001 Westonia 100 100 100 100
#> last24_hours last7_days_since9_am last7_days_since12_am
#> <int> <int> <int>
#> 1: 100 100 100
#> last14_days_since9_am last14_days_since12_am month_to_date_to9_am
#> <int> <int> <int>
#> 1: 100 100 100
#> month_to_date_since12_am year_to_date_to9_am year_to_date_since12_am
#> <int> <int> <num>
#> 1: 100 100 99.9
Example 15: Checking Station Availability for a Set Time Period
Check the availability of the Binnu station for January of 2018. When
a custom start_date
is provided an end_date
must also be provided.
library(weatherOz)
(
BI_201801 <- get_dpird_availability(
station_code = "BI",
start_date = "2018-01-01",
end_date = "2018-01-31"
)
)
#> Key: <station_code>
#> station_code station_name start_date end_date availability_since_9_am
#> <fctr> <char> <POSc> <POSc> <int>
#> 1: BI Binnu 2018-01-01 2018-01-31 100
#> availability_since_12_am
#> <int>
#> 1: 100
Getting Station Metadata for the DPIRD Network Stations
The get_stations_metadata()
function is shared with the
SILO functions as well, so this function will retrieve data from both
weather APIs. Shown here is how to use it for DPIRD data only and with
an example of DPIRD specific information, namely including closed
stations and rich metadata.
Example 16: Get DPIRD Station Metadata
The get_stations_metadata()
function allows you to get
details about the stations themselves for stations in the DPIRD and SILO
(BOM) networks in one function. Here we demonstrate how to get the
metadata for the DPIRD stations only.
library(weatherOz)
(metadata <- get_stations_metadata(which_api = "dpird"))
#> station_code station_name start end latitude longitude
#> <char> <char> <Date> <Date> <num> <num>
#> 1: AN001 Allanooka 2012-06-19 2024-11-17 -29.06361 114.9972
#> 2: AM001 Amelup 2019-10-09 2024-11-17 -34.27083 118.2685
#> 3: SH002 Babakin 2016-06-22 2024-11-17 -32.12548 118.0041
#> 4: BA Badgingarra 2008-11-19 2024-11-17 -30.33805 115.5395
#> 5: BP001 Balingup 2014-10-24 2024-11-17 -33.79620 116.0640
#> ---
#> 220: YS Yilgarn 2008-11-01 2024-11-17 -31.91562 119.2561
#> 221: YE001 York East 2013-11-08 2024-11-17 -31.83588 116.9211
#> 222: YU001 Yuna 2012-06-21 2024-11-17 -28.33763 114.9898
#> 223: YU002 Yuna NE 2016-03-24 2024-11-17 -28.20032 115.2616
#> 224: YU003 Yuna North 2018-08-08 2024-11-17 -28.12086 114.9626
#> state elev_m
#> <char> <int>
#> 1: WA 131
#> 2: WA 200
#> 3: WA 313
#> 4: WA 284
#> 5: WA 227
#> ---
#> 220: WA 468
#> 221: WA 229
#> 222: WA 329
#> 223: WA 267
#> 224: WA 264
#> source
#> <char>
#> 1: WA Department of Primary Industries and Regional Development (DPIRD)
#> 2: WA Department of Primary Industries and Regional Development (DPIRD)
#> 3: WA Department of Primary Industries and Regional Development (DPIRD)
#> 4: WA Department of Primary Industries and Regional Development (DPIRD)
#> 5: WA Department of Primary Industries and Regional Development (DPIRD)
#> ---
#> 220: WA Department of Primary Industries and Regional Development (DPIRD)
#> 221: WA Department of Primary Industries and Regional Development (DPIRD)
#> 222: WA Department of Primary Industries and Regional Development (DPIRD)
#> 223: WA Department of Primary Industries and Regional Development (DPIRD)
#> 224: WA Department of Primary Industries and Regional Development (DPIRD)
#> status wmo
#> <char> <lgcl>
#> 1: open NA
#> 2: open NA
#> 3: open NA
#> 4: open NA
#> 5: open NA
#> ---
#> 220: open NA
#> 221: open NA
#> 222: open NA
#> 223: open NA
#> 224: open NA
Example 17: Get Rich DPIRD Station Metadata and Include Closed Stations
You can fetch additional information about the DPIRD stations as well
as getting data for stations that are no longer open like so with the
rich
and include_closed
arguments set to
TRUE
.
library(weatherOz)
(metadata <- get_stations_metadata(which_api = "dpird",
include_closed = TRUE,
rich = TRUE))
#> station_code station_name start end latitude longitude
#> <char> <char> <Date> <Date> <num> <num>
#> 1: AN001 Allanooka 2012-06-19 2024-11-17 -29.06361 114.9972
#> 2: AM001 Amelup 2019-10-09 2024-11-17 -34.27083 118.2685
#> 3: SH002 Babakin 2016-06-22 2024-11-17 -32.12548 118.0041
#> 4: BA Badgingarra 2008-11-19 2024-11-17 -30.33805 115.5395
#> 5: BP001 Balingup 2014-10-24 2024-11-17 -33.79620 116.0640
#> ---
#> 235: YS Yilgarn 2008-11-01 2024-11-17 -31.91562 119.2561
#> 236: YE001 York East 2013-11-08 2024-11-17 -31.83588 116.9211
#> 237: YU001 Yuna 2012-06-21 2024-11-17 -28.33763 114.9898
#> 238: YU002 Yuna NE 2016-03-24 2024-11-17 -28.20032 115.2616
#> 239: YU003 Yuna North 2018-08-08 2024-11-17 -28.12086 114.9626
#> state elev_m
#> <char> <int>
#> 1: WA 131
#> 2: WA 200
#> 3: WA 313
#> 4: WA 284
#> 5: WA 227
#> ---
#> 235: WA 468
#> 236: WA 229
#> 237: WA 329
#> 238: WA 267
#> 239: WA 264
#> source
#> <char>
#> 1: WA Department of Primary Industries and Regional Development (DPIRD)
#> 2: WA Department of Primary Industries and Regional Development (DPIRD)
#> 3: WA Department of Primary Industries and Regional Development (DPIRD)
#> 4: WA Department of Primary Industries and Regional Development (DPIRD)
#> 5: WA Department of Primary Industries and Regional Development (DPIRD)
#> ---
#> 235: WA Department of Primary Industries and Regional Development (DPIRD)
#> 236: WA Department of Primary Industries and Regional Development (DPIRD)
#> 237: WA Department of Primary Industries and Regional Development (DPIRD)
#> 238: WA Department of Primary Industries and Regional Development (DPIRD)
#> 239: WA Department of Primary Industries and Regional Development (DPIRD)
#> status wmo probe_height rain_gauge_height wind_probe_heights
#> <char> <lgcl> <num> <num> <list>
#> 1: open NA 1.25 0.5 3
#> 2: open NA 1.25 1.0 3
#> 3: open NA 1.25 0.5 3
#> 4: open NA 1.25 0.5 3
#> 5: open NA 1.25 0.5 3
#> ---
#> 235: open NA 1.25 0.5 3
#> 236: open NA 1.25 0.5 3
#> 237: open NA 1.25 0.5 3
#> 238: open NA 1.25 0.5 3
#> 239: open NA 1.25 0.5 3
#> air_temperature battery_voltage delta_t dew_point pan_evaporation
#> <lgcl> <lgcl> <lgcl> <lgcl> <lgcl>
#> 1: TRUE TRUE TRUE TRUE TRUE
#> 2: TRUE TRUE TRUE TRUE TRUE
#> 3: TRUE TRUE TRUE TRUE TRUE
#> 4: TRUE TRUE TRUE TRUE TRUE
#> 5: TRUE TRUE TRUE TRUE TRUE
#> ---
#> 235: TRUE TRUE TRUE TRUE TRUE
#> 236: TRUE TRUE TRUE TRUE TRUE
#> 237: TRUE TRUE TRUE TRUE TRUE
#> 238: TRUE TRUE TRUE TRUE TRUE
#> 239: TRUE TRUE TRUE TRUE TRUE
#> relative_humidity barometric_pressure rainfall soil_temperature
#> <lgcl> <lgcl> <lgcl> <lgcl>
#> 1: TRUE FALSE TRUE FALSE
#> 2: TRUE FALSE TRUE FALSE
#> 3: TRUE FALSE TRUE TRUE
#> 4: TRUE FALSE TRUE TRUE
#> 5: TRUE FALSE TRUE TRUE
#> ---
#> 235: TRUE FALSE TRUE TRUE
#> 236: TRUE FALSE TRUE TRUE
#> 237: TRUE FALSE TRUE FALSE
#> 238: TRUE FALSE TRUE TRUE
#> 239: TRUE FALSE TRUE TRUE
#> solar_irradiance wet_bulb wind1 wind2 wind3 apparent_temperature
#> <lgcl> <lgcl> <lgcl> <lgcl> <lgcl> <lgcl>
#> 1: TRUE TRUE TRUE FALSE FALSE TRUE
#> 2: TRUE TRUE TRUE FALSE FALSE TRUE
#> 3: TRUE TRUE TRUE FALSE FALSE TRUE
#> 4: TRUE TRUE TRUE FALSE FALSE TRUE
#> 5: TRUE TRUE TRUE FALSE FALSE TRUE
#> ---
#> 235: TRUE TRUE TRUE FALSE FALSE TRUE
#> 236: TRUE TRUE TRUE FALSE FALSE TRUE
#> 237: TRUE TRUE TRUE FALSE FALSE TRUE
#> 238: TRUE TRUE TRUE FALSE FALSE TRUE
#> 239: TRUE TRUE TRUE FALSE FALSE TRUE
#> eto_short eto_tall frost_condition heat_condition wind_erosion_condition
#> <lgcl> <lgcl> <lgcl> <lgcl> <lgcl>
#> 1: TRUE TRUE TRUE TRUE TRUE
#> 2: TRUE TRUE TRUE TRUE TRUE
#> 3: TRUE TRUE TRUE TRUE TRUE
#> 4: TRUE TRUE TRUE TRUE TRUE
#> 5: TRUE TRUE TRUE TRUE TRUE
#> ---
#> 235: TRUE TRUE TRUE TRUE TRUE
#> 236: TRUE TRUE TRUE TRUE TRUE
#> 237: TRUE TRUE TRUE TRUE TRUE
#> 238: TRUE TRUE TRUE TRUE TRUE
#> 239: TRUE TRUE TRUE TRUE TRUE
#> richardson_unit chill_hour
#> <lgcl> <lgcl>
#> 1: TRUE TRUE
#> 2: TRUE TRUE
#> 3: TRUE TRUE
#> 4: TRUE TRUE
#> 5: TRUE TRUE
#> ---
#> 235: TRUE TRUE
#> 236: TRUE TRUE
#> 237: TRUE TRUE
#> 238: TRUE TRUE
#> 239: TRUE TRUE